When hiring a .NET developer, the least you should do is waste time with a scattered, unorganized approach. Instead, you can focus on asking the right questions to ensure you hire the right person for the .NET developer role. More often than not, using the wrong resources puts employers at risk of slowing down the hiring process and wasting time and money.
We discuss interviewing in-depth with Alexandra Țigău-Almăşan, a Senior Fullstack Engineer at Proxify, and other professionals who have worked closely with .NET developers. She carefully helped us formulate a go-to checklist for interviewing .NET developer candidates.
The interview questions and answers
To make everything as concise and relevant as possible, we rounded up the 10 essential questions that guarantee a successful interview. For a successful interview, you conduct the process so that candidates understand what is asked of them. This allows them to answer all questions timely and effectively, potentially placing themselves among the shortlisted candidates.
1. Explaining how “async await” works in .NET
Here, it’s best to provide a code snippet for the candidate to review and give their opinion. The candidate should be able to tell us the order in which the messages will be displayed on the screen at runtime.
This code snippet below is a ready-to-use example for candidates, for which they need to provide the answer:
static void Main()
{
Task task = new Task(ReadFileMethod);
task.Start();
task.Wait();
}
static async void ReadFileMethod()
{
var filePath = "C:\\text.txt";
var length = await ReadFile(filePath);
Console.WriteLine(" Total length: " + length);
}
static async Task<int> ReadFile(string file)
{
var length = 0;
using (StreamReader reader = new StreamReader(file))
{
var s = await reader.ReadToEndAsync();
length = s.Length;
}
return length;
}
2. Explaining the difference between IQueryable and IEnumerable
This question is fundamental because it shows how well a candidate resonates with the basics.
One of these work better than the other since filters are applied to the database (IQueryable), and the other one takes data from the database only after it filters it. This data filtering leads to many elements in memory which we may not need.
Besides this, the candidate's answer can contain other information, like telling us when it would be useful to use IEnumerable, which is useful when filtering data we already have in memory. At the same time, the IQueryable is useful when using Linq for SQL queries.
3. Explaining the differences between “dispose” and “finalize”
Maybe not many people heard of the two ways to free up unmanaged resources, so let’s cover them too.
The main difference is that we have to call dispose in the code, whereas, for the finalize method, we need to call it automatically through the garbage collection. If the candidate likes to give examples, it is best to receive an answer explaining how and why we use dispose when we have a close() method call. For example, we use it when we stop reading a file or a stream, and at the same time, the other method exists automatically when we are finishing a using statement.
4. Testing how a candidate provides solutions to possible issues
“Imagine that we have an application that sends various emails synchronously, and after a while, developers find out that users wait too long to receive emails – this is a usual situation where many users try to perform this action at once. Ask candidates how they would resolve this issue. This scenario is useful because it makes candidates think of the project's scalability.”
There are multiple valid solutions to this, and the simplest one would be to say that we make the email-sending process async, and we are adding every email that needs to be sent to a queue. This queue can be monitored, and if something goes wrong, we can easily see what it is that’s not working, so retrying is possible and easy to do.
5. Explaining when and why we use reflection and which feature they see in a .NET code sample
We developers often want to inspect assemblies or check what we have done so far with reflection. Besides the assemblies, we can also see information about types, members, methods, and even test parts of the code dependent on a private class.
Although, there is one disadvantage to using reflection. It would be nice to see if the candidate knows about the disadvantage, such as whether using reflection is slower than relying on direct calls. And also, using reflection means removing type safety that the compiler enforces.
Here is an example of reflection – in this case, a candidate should themselves recognize that a code snippet is reflection, and say more about it afterwards:
string aString = "this is a string";
var type = aString.GetType();
6. Revising code samples
“Since .NET always adds new features, the candidate should know about records and how they work. We would like to see how well a candidate understands immutability, meaning we cannot change the object after the object is created. In this case, we cannot change the employee’s name on the third line, as shown in the code sample below. Also, if the candidate knows how to fix this immutability, that is a great plus.”
record Employee(int Id, string Name, int Age);
var employee = new Employee(1, “John Doe”, 25);
employee.Name = “John D. Doe”;
And the solution to immutability you could expect:
employee = employee with {Name = “Mark Doe”};
7. Explaining what to do to increase an app’s security
Many things can be done in a project to contribute to better security of the app at hand. Some of them are:
-
Encrypting of data
-
Avoiding connection strings and other passwords added in the settings files
-
Using authorization on all API endpoints
-
Keeping packages we use up to date (these usually fix security issues when updates are made).
Answers that offer real-life solutions to real-life issues are most appreciated because they are practical. Also, look for answers that explain exactly how the issue was resolved.
8. Asking about memory management of .NET
“Developers always need to be careful with how they manage memory and ensure that there are no memory leakages anywhere. Also, they need to understand what .NET does in this memory context and ensure that they dispose of everything from memory at the right time. If the candidate knows about garbage collectors, the states of memory, and how the memory is allocated and deallocated, this is a big advantage.”
9. Explaining the DDD (domain-driven design) and its usage
For example, a candidate here should know that DDD implies to some event happening in the domain and that other parts of the domain should “know” of this and possibly do other actions due to this event.
Candidates get extra points for knowing the drawbacks of DDD, such as not respecting certain SOLID principles and listing them precisely. All of this will show you how well a candidate would achieve this when coding an app.
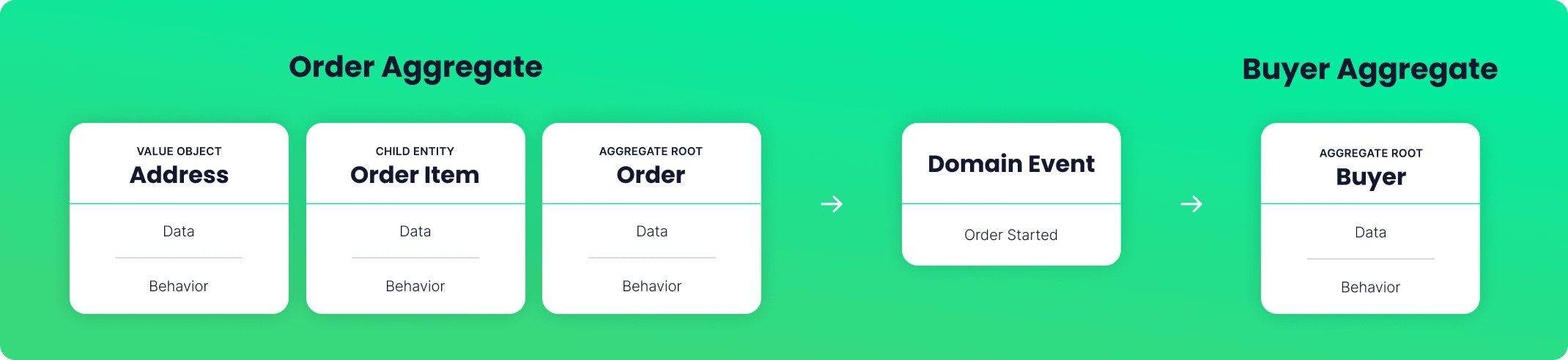
10. Explaining the meaning and usage of SQL query
Let’s say we have a chart table with product information like ID, name, quantity, and price for certain products.
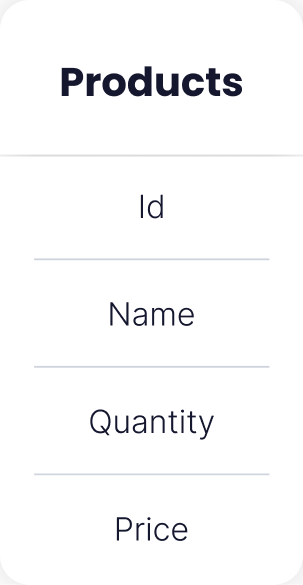
Candidates should answer next: what exactly does the following query do?
SELECT Name
FROM Products A
WHERE (
SELECT COUNT(*)
FROM Products B
WHERE B.Name = A.Name
) > 1;
The query returns all the duplicate product names from the list, but it doesn’t do it optimally. Candidates here should figure out how to achieve the optimal version, as shown below:
SELECT Name
FROM Products
GROUP BY Name
HAVING COUNT(*) > 1;
And, regardless of the programming language used, for a .NET developer it’s essential to know how to create and read basic SQL scripts like these.
Refer to a checklist for the hard and soft skills too
Now, you have a list of the essential questions to ask but to make the interview even more valuable, you’d also need to use a checklist of hard and soft skills hand in hand.
When you refer to hard skills, you confirm whether a candidate answered everything correctly and you tick off the needed technical knowledge requirements. And when you do this with soft skills too, you value candidates’ personality and communication, and you see potential in it to fit with your team and company.
Hard skills
A .NET candidate has exceptional knowledge of this framework if they meet most / all of the following requirements in the hard skills list:
-
A degree in engineering, computer science, or similar related fields relevant to the job
-
More than three years of experience with .NET / backend
-
Excellent knowledge of at least one or two .NET programming languages (C#, Visual Basic, F#)
-
Knowledge of ASP.NET and SQL Server
-
Experience working with design/architectural patterns like MVC (model-view-controller)
-
Good understanding of Agile
-
Experience with APIs b architectures; RPC and REST.
“A candidate should master the SOLID principles, too, as well as SQL. The troubleshooting skills should be excellent, and the code should be written in a way that is easy to maintain and scale. Lastly, they must be good at debugging and always keep up with the latest .NET updates.”
How AI is shaping the future of programming
Discover the responses of developers from our network about their use of AI in their development work, the benefits and challenges they face, and their predictions for the future of AI in software development.
Soft skills
A successful hire won’t be complete unless the shortlisted candidate shows excellent soft skills. They can be as professional and experienced as you’d want them to be, but you have to think a few steps ahead – would you want someone uncooperative and too individualistic in your team?
Notice if candidates:
-
Use good English
-
Take care of a professional look (their room and themselves)
-
Listen carefully
-
Exhibit curiosity for the role
-
Show team player qualities
-
Show overall punctuality including for their task answers too
-
Have a problem-solving mindset
“When a developer is curious about the latest .NET updates and is well-informed, this tells you they are not satisfied with the status quo and always progress their skills. A great developer will always seek more knowledge of new .NET versions. For example, they’d know that the .NET framework changed to .NET Core, or whatever they know and find out, they’d share it if it’s useful to them and the team.”
Why do you need a good .NET developer?
Hiring a good .NET developer will soon show you why it pays off. For example, they will:
- Write quality code with .NET languages
- Upgrade, configure, and debug systems
- Improve portability
- Provide support for any desktop, mobile, and web application
- Improve maintainability
- Significantly improve the existing software
- Manage extra-fast development
- Achieve top-notch security
- Boost scalability
The benefits of .NET are substantial in the long run and too important to ignore. Ataur Rahman, founder and CEO of GetGenie, generously shared a few essential benefits of hiring .NET developers,
-
Robust app development – Good .NET developers have a deep knowledge of this framework. Thus, they can build scalable and robust applications to cater to business needs. This also allows them to handle complex data processing continuously.
-
Cross-platform compatibility – One notable strength of .NET developers is creating performant cross-platform apps running on multiple devices and operating systems. Organizations and companies can expand their reach and ensure a consistent user experience across different platforms.
-
Seamless integration with existing systems – With a rich experience in integrating disparate systems, .NET developers can connect your company’s technology infrastructure by facilitating efficient data exchange and improving operational efficiency.
-
Access to a broad community – This framework boasts a vast and broadly rich network of developers, offering access to a wealth of libraries, resources, and best practices helpful to everyone. So, hiring a .NET developer will allow your business to tap into this rich knowledge base, enabling much faster development cycles in the future and more effortless, smoother troubleshooting.
There is another point of view you want to look at, coming from Norbert Raus, co-founder and CEO of Alphamoon. In his distinctive way, he doesn’t downplay the quality of a good .NET developer and what this can do for your budget.
“It wouldn’t be too outlandish to say that a .NET developer can build you ANY app you want – from the interactive web and AI-powered apps, cloud-based SaaS solutions, to native and cross-platform mobile apps. It’s like buying one thing and getting many through cross-platform compatibility that optimizes your software development budget.”
Making the interview more relevant
When interviewing for the next .NET developer, some things might help you make the process concise and relevant.
First, you need to ensure that everything is streamlined and well-organized to anticipate recruitment challenges ahead of time.
As Alexandra explains, knowing the differences in the level of expertise is another significant factor in all of this. Usually, the Senior developers are good at thinking a few steps ahead, but that doesn’t mean that medior ones lack knowledge.
“What helps you differentiate between senior, junior, or mid developer is knowing that code will work well in a current situation and what code would become obsolete. Also, with more years of experience, there are likely fewer errors in the code and better code performance. A more experienced .NET developer will also cover more edge case scenarios that would otherwise have to be discovered after the feature/task was implemented.”
The co-founder and CEO of SocialPlug, Mark Voronov, didn’t hold back from providing his insightful point of view. As an entrepreneur working closely with .NET developers, it wasn’t difficult for him to describe what makes a developer stand out in a large pool of candidates. His advice for conducting a relevant interview is to look for someone who is a team player just as much as they are curious and experienced.
“When evaluating .NET developer candidates, several key qualities must be considered. Look for those with a strong technical .NET foundation and a passion for continuously learning and keeping up with industry trends. They will think critically, solve complex problems, and communicate effectively within a team. Also, they’ll have a track record of successful projects and adaptability–with a collaborative mindset; they’ll greatly impact your development team.”
Overall, cover the blend of all skills, not just the technical ones. You should also look for soft skills, communication styles, rapport speed, and other factors that make a candidate a valuable asset to the team.