Builders: The podcast where we discuss the ups and downs of building great tech products Stream here
Hire senior and proven JavaScript developers
Gain access to 3000+ experts, available to start work immediately.
Discover the top 2% who have passed extensive assessments.
Hire JavaScript developers without additional employment fees or overheads.
Partner with a personal matcher and find JavaScript developers that fit your needs.
Find a JavaScript developer
Get StartedFind a JavaScript developer
Get StartedHire fast with Proxify
Hire JavaScript developers, fast
We know that finding the perfect JavaScript developer can be time-consuming and expensive. That's why we've created a solution that saves you time and money in the long run.
Our JavaScript developers are vetted and tested for their technical skills, English language proficiency, and culture fit aspects to ensure that we provide you with the perfect match for your engagement. With our hiring experts, you can easily discuss any issues, concerns, or onboarding processes and start your engagement quickly.
Our JavaScript developers are also skilled in a diverse range of additional frameworks and tools, meaning you find the right candidate for your business needs, committed to delivering outstanding results, always.
Talented JavaScript developers available now
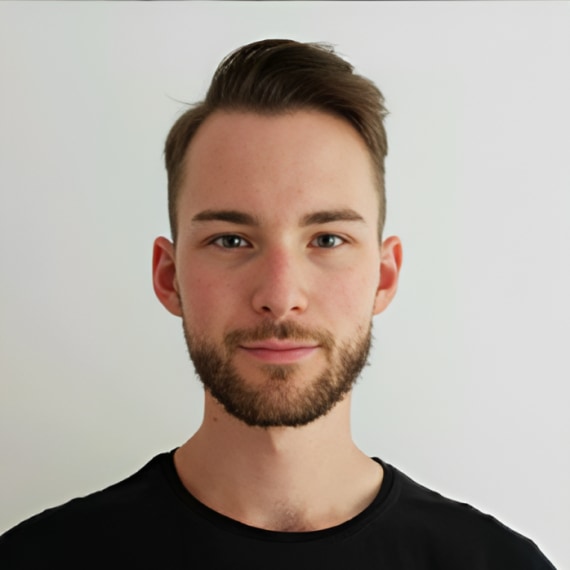
Amir M.
Bosnia and Herzegovina
Fullstack Developer
5 years experience
Verified member
Expert in
- JavaScript
- Amazon CloudWatch
- AWS Lambda
- AWS S3
- AWS SQS
- +36
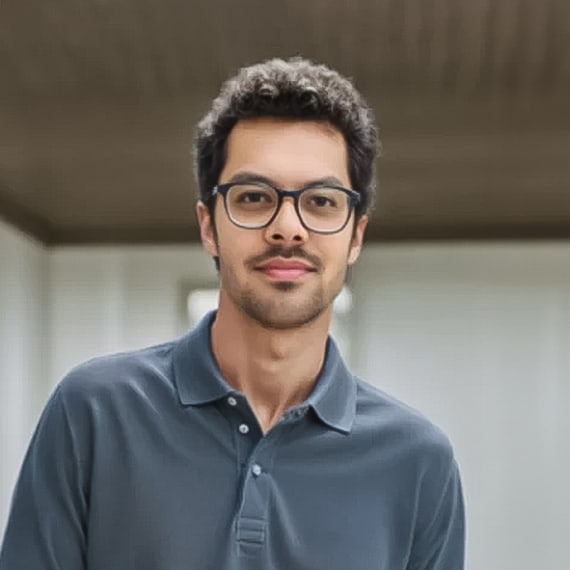
Jerome V.
Brazil
Backend Developer
10 years experience
Verified member
Expert in
- JavaScript
- Node.js
- AWS
- C
- MongoDB
- +15
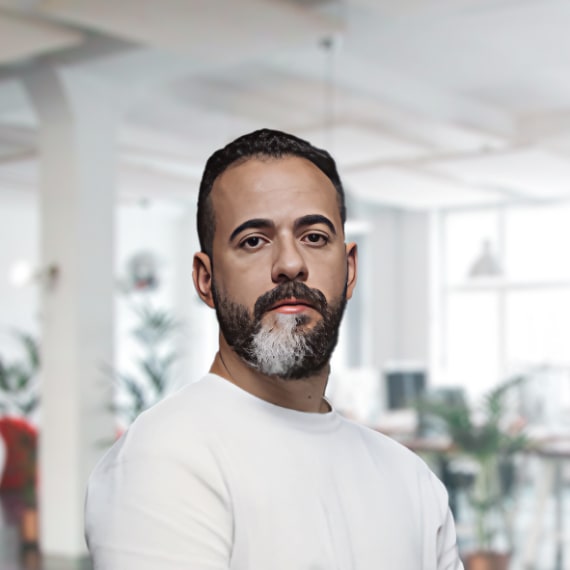
Aldo T.
Albania
React.js Developer
6 years experience
Trusted member since 2021
Expert in
- JavaScript
- React.js
- HTML / CSS
- Redux.js
- Bootstrap
- +3
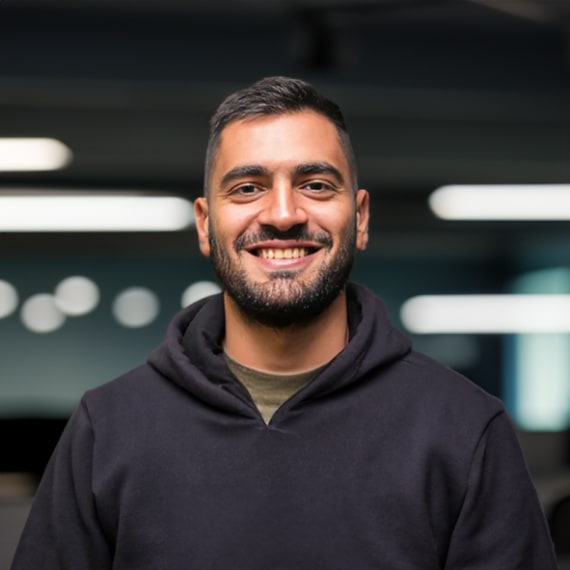
Tomas N.
Argentina
Fullstack Developer
5 years experience
Trusted member since 2022
Expert in
- JavaScript
- React.js
- Node.js
- Git
- MongoDB
- +18
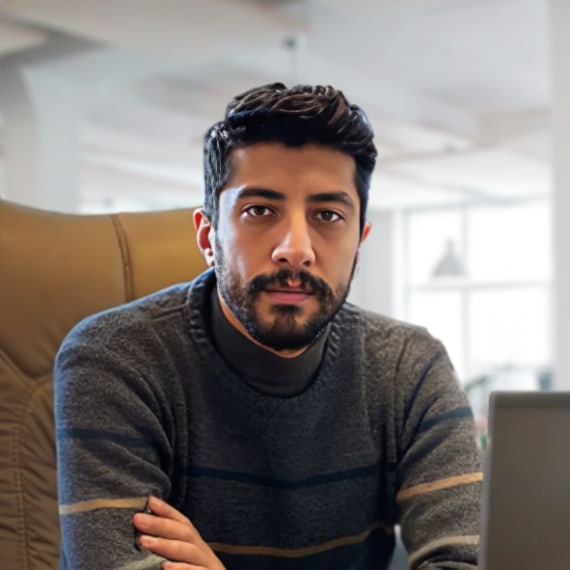
Amin Y.
Turkey
Fullstack Developer
5 years experience
Verified member
Expert in
- JavaScript
- Docker
- Express.js
- HTML / CSS
- MongoDB
- +12
A complete hiring guide for JavaScript Developers in 2024
Introduced in 1995 as part of the then-popular browser Netscape Navigator, JavaScript is a lightweight interpreted programming language that treats functions as first-class citizens. Its creator, Brendan Eich, initially conceived it to help non-programmers create dynamic, interactive websites. However, JavaScript has evolved into a powerful language capable of building complex web, mobile, and server-side applications.
One of the many reasons for its popularity is its versatility and accessibility. It runs natively on all modern web browsers and servers (using the Node.js runtime environment), making it the only language versatile enough to be used for both frontend and backend development. Apart from this, it has a strong ecosystem of libraries and frameworks, making it a powerful tool for developers of all levels.
Some of its key features are:
- First-class functions: Functions in JavaScript are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions. This allows for a high degree of flexibility in coding.
- Prototypal inheritance: JavaScript's object model is based on prototypes rather than classical inheritance, providing a dynamic and flexible means of object creation and inheritance.
- Closures: JavaScript supports closures, which allow functions to retain access to their lexical scope even when invoked outside of that scope. This feature is crucial for creating private variables and functions.
Industries and applications
JavaScript is the exclusive scripting language for all major web browsers, so it is ever-present in all modern websites, enabling the creation of dynamic and interactive web applications. Frameworks like Angular, Vue.js, and React have revolutionized web development by facilitating the creation of single-page applications that offer a seamless, app-like experience. JavaScript is indispensable in the modern digital world, from powering dynamic shopping experiences to building instant feeds for social media platforms to ensuring safe and secure online banking.
Runtimes such as Node.js have also extended JavaScript’s capabilities to server-side development. This unification has allowed developers to use the same language for client- and server-side applications, streamlining the development process.
Must-have technical skills
When hiring JavaScript developers, looking beyond their ability to write code is essential. These skills ensure they can tackle real-world projects effectively.
- JavaScript syntax and core language concepts: Proficiency in JavaScript syntax and a solid grasp of core concepts such as functions as data, lexical scoping, prototype chains, and the event loop are fundamental skills required to become a JavaScript developer.
- Object-oriented programming or functional programming: JavaScript supports both object-oriented and functional programming paradigms. A skilled JavaScript developer is expected to grasp either object-oriented programming (OOP) or functional programming well.
- Web development and RESTful APIs: JavaScript developers are often required to build and maintain web applications using frameworks like React, Angular, or Express.js. Understanding the fundamentals of web development, including the ability to work with RESTful APIs, is essential to being a practically skilled JavaScript developer.
- Asynchronous programming: Understanding asynchronous programming concepts in JavaScript is crucial, especially event handling and promises. Promises have become the de facto way of handling asynchronous operations, replacing callbacks for cleaner and more manageable code.
- Database and storage solutions: Real-world applications require developers to work with storage solutions such as PostgreSQL, MongoDB, Redis, etc. Proficient JavaScript developers are also expected to be proficient in some storage technologies, especially if they desire to build server-side applications.
Nice-to-have technical skills
Beyond the essentials, nice-to-have skills can make a JavaScript developer truly shine. These include:
- Test-driven development (TDD): This is a style of development in which the developer first writes the test cases and then implements the business logic to make the tests pass. You should read more about it in this article by Martin Fowler
- Version control: Familiarity with version control tools such as Git is a valuable skill to have to collaborate better. Besides collaboration, version control also simplifies code management, distribution, debugging, etc.
- Advanced JavaScript techniques: Understanding lexical scoping and efficiently using functions as data can help developers solve complex problems elegantly.
- TypeScript: TypeScript is a superset of JavaScript that adds type abilities to JavaScript. TypeScript is helpful in catching errors at compile time, improving code quality, and making large codebases easier to manage. Therefore, a developer's understanding of TypeScript is beneficial.
- GraphQL and WebSockets: WebSockets and GraphQL are essential for JavaScript developers to master due to their ability to provide real-time communication, efficient data handling, and a better overall user experience. These technologies represent key advancements in web development, offering significant improvements over traditional methods.
Interview questions and answers (coding questions)
1. What is the difference between let
and const
in JavaScript?
Example answer: Variables defined using const
cannot be modified, while there is no such restriction on variables defined using let.
For example:
const a = 2;
a = 4; // will throw error
let a = 4;
a = 3; // works
2. Given the following code, when do you expect the message "hello, there" to be printed on your console?
setTimeout(() => console.log("hello, there"), 500);
const t1 = Date.now() // current unix timestamp in ms
while (true) {
if (Date.now() - t1 > 10000) {
// Break out of the loop after 10 seconds
break;
}
}
Example answer: The message "hello, there" will be printed after 10 seconds even though the timeout callback function is scheduled to run after 500 ms. This happens because JavaScript runs on a single thread called the event loop. The function that prints the message "hello, there" is added to the event queue almost after 500 ms. However, since the event loop is busy processing the while
loop, the event loop can execute the function in the callback queue only after 10 seconds.
3. How can you use a promise to implement a sleep
function in JavaScript?
Example answer: The sleep
function would return a promise, resolving only after the desired time. The implementation of the function would be:
function sleep(timeMs) {
return new Promise((resolve, reject) => {
setTimeout(() => resolve(), timeMs);
});
}
In the above function, we return a promise. The promise is only resolved after the given timeMs. The function can be used by either await
-ing the returned promise, or using a then
chain. Example of using it in a then
chain:
sleep(1000).then(() => console.log('This should be printed after 1000 ms'));
4. How does JavaScript manage the memory usage of your program?
Example answer: JavaScript uses a mark-and-sweep garbage collection approach to manage memory. The basic idea of this algorithm is to find all reachable objects, starting from the global object and determining the set of unreachable objects. The unreachable objects are then cleared out from memory.
5. How does JavaScript handle asynchronous operations internally?
Example answer: Most asynchronous operations, such as I/O operations or timer-based operations (setTimeout
) is provided by the web APIs (in the case of browsers) or the libuv
APIs (in the case of Node.js). These asynchronous tasks are offloaded to the respective APIs, which are responsible for queueing the callback functions in the callback queue. The event loop then executes these callback functions one by one once its call stack is empty and it has nothing else to execute.
6. What are JavaScript generators, and how are they helpful?
Example answer: Generator functions are special functions whose execution can be paused and resumed. Unlike normal functions, they do not follow the run-to-completion model. Generators return an iterator that adheres to the iterable protocol. The function execution is paused using the yield
keyword which yields the defined value to the calling function and pauses the generator function's execution until the next()
method is called on the returned iterator.
Since generators enable lazy evaluations, they can be used in cases where we want to theoretically run an infinite loop that continuously generates positive integers as many as required.
function* idGenerator() {
let id = 0;
while (true) {
yield ++id;
}
}
const gen = idGenerator();
console.log(gen.next().value); // 0
console.log(gen.next().value); // 1
console.log(gen.next().value); // 2
// and so on…
7. What is the difference between ===
(triple equals) comparison and same-value-zero equality?
Example answer: The triple-equals operator (also called the strict equality operator) compares the operators' types and values. So,' 2'!== 2.
However, this operator returns false information about any one of the operands in NaN
. In fact, one could say that x !== x
when x = NaN.
Same-value-zero equality is the same as the strict equality operator (triple equals), except that it treats NaN
as a single object, and hence, comparing NaN
with NaN
returns true. JavaScript does not directly expose any API for this comparison, but it can be implemented as a function. The function would essentially return the result of the strict equality comparison. But before this comparison, it can early return true if both operands are NaN
(using isNaN()
).
8. Assuming that an object (person
) is defined with two properties: name
and age
, how can you ensure that the property age
always returns a Numeric value even if it is stored as a string? Use your knowledge of JavaScript’s Proxy object to achieve the desired result.
Example answer: A get
trap can be defined as one that intercepts all attempts to access any properties of the object. Once intercepted, any custom logic can be used to return custom values. Example usage:
const person = {
name: "Alice",
age: "30",
};
const handler = {
get: function(target, prop) {
if (prop === 'age') {
return Number(target[prop]);
}
return target[prop];
}
}
const proxiedPerson = new Proxy(person, handler);
console.log(proxiedPerson.age); // 30 (integer)
9. Create a function createCounter
that returns another function increment.
Every time the returned function is called, it returns a new number that is 1 greater than the previous number returned. There should be no way to directly access or modify the internal counter of createCounter
. The expectation is the following:
const increment = createCounter();
increment() // 1
increment() // 2
increment() // 3
Example answer: The function createCounter
would maintain an internal variable, count
, which holds the current count. Each time the increment
function is called, the variable's value (count
) will be increased by 1 and returned.
function createCounter() {
let count = 0;
return () => ++count;
}
As can be seen, the count variable cannot be read or modified directly from outside the createCounter function.
10. Given the following code snippet, what will be the output of the greet
and person.greet
calls? Provide explanations for your answer.
const person = {
name: 'Alice',
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
const greet = person.greet;
greet(); // What is the output?
person.greet(); // What is the output?
Example answer: Output would be:
greet(); // Hello, my name is undefined
person.greet(); // Hello, my name is Alice
The greet
function on its own is bound to the global context, while person.greet
is bound to the person
context. This means that in case greet, this
refers to the global object that does not have the name
property defined. But in the case of a person.greet
, this refers to a person
, which has the name
property defined.
Interview questions and answers (technical understanding)
Use the following questions and their example answers when you assess JavaScript developer candidates.
1. Can you define isNaN and its usage?
Example answer: NaN means "not a number," and isNaN lets us know which value is, in fact, NaN. When we use the function isNaN(), we try to discover if a value has an incorrect or illegal number. If this function returns as 'true' the examined value is NaN.
2. Can you define "negative infinity"?
Example answer: If we divide a negative number by zero, we get a negative infinity number in JavaScript. This number is a constant value, the lowest we can receive, and there is no lower value than this.
3. Could you explain the "global variables "?
Example answer: Global variables can be accessed from anywhere in the program. Global variables are always declared via a window object or outside a function. We can access them from any other function. An example of a global variable is var.
4. Can you differentiate between SessionState and ViewState in JavaScript?
Example answer: The SessionState is data that persists in a specific server. When we close the browser, or a session expires, this data availability also stops. We can access this precise data from all web app pages.
The ViewState is a group of controls' values on a page and the client browser. ViewState stores information only on the client's side.
The SessionState is saved on a server, but the ViewState is saved on the page itself, and we can see it from just a single page, not more.
5. Can you define the "looping structures" in JavaScript?
Example answer: We use these looping structures when we work on repetitive tasks, i.e., this feature helps us to repeat functions. The loops don't stop running unless their condition appears "false.”
There are seven looping structures in JavaScript:
- for…in
- for…of
- do-while
- while
- for
- forEach()
- map()
6. Define "null" in JavaScript?
Example answer: "null' means either no object or no value. The other name for this is null string, which intentionally has no value. We call this a primitive value because it has no array object.
For context, the primitive values can be divided into:
- Number
- Boolean
- String
- Null
- Undefined
7. Can you explain how to use 'Void(0)'?
Example answer: 'Void' in JavaScript represents 'return of nothing,' an empty value. So, when we use 'Void(0), we call a different method without refreshing the page. Further, when we use "javascript:void(0)," we always do this in an anchor tag, and this prevents reloading of the page. Also, we can call the JavaScript functions on a single or double click this way.
8. Can you explain more about the 'pop()' method?
Example answer: We use this method when we need to remove the last array element, i.e., pop() helps us retrieve that same element once someone removes it. This way, we adjust the array length accordingly with the help of the syntax array.pop()
9. Can you differentiate between .call() and .apply()?
Example answer: These two are similar to one another. If we know some function's arguments and the function number, we use .call() as a predefined method. With this method, a specific object can take on another method from a different object.
On the other hand, we use .apply() if we don't know the function number, but we can write a method we can later use on more different objects.
Industries where JavaScript is commonly used
JavaScript has versatile uses, but it is most widely used and famous for:
Finances
Many companies for payment processing and finances use JavaScript for their website's frontend. Payment apps made with JavaScript provide practical features for the financial sector of that business.
The apps are scalable, quick, and highly performative, crucial for payment processing businesses or anything financial-related.
Marketing and advertising
With JavaScript, it is easy to create visually appealing interactive effects that are the core of any good marketing relying on visuals. With the help of Google Analytics, JavaScript developers explore the advertising market and ensure a good user experience and successful ROI.
Healthcare
JavaScript developers efficiently work on the healthcare apps' data and its protection. They also work on the frontend of these apps to make them as simple as possible and maximally effective for all their users. One example is the healthcare billing apps, or apps for scheduling appointments.
eCommerce
In eCommerce, the user-friendly experience is more important than anything else. JavaScript developers efficiently work on simple app navigation so users can work through an app quickly (e.g., adding items to the shopping cart or arriving at checkout & payment sections).
Social media platforms
Social media platforms rely on JavaScript for both the frontend and backend. Also, developers know that if they disable JavaScript on a browser, the user won't be able to log into a particular social media account – all of this emphasizes how important JavaScript is for developers and users.
Business benefits of using JavaScript
This is why you need JavaScript for your business:
It's cost-efficient
Every business wants to save money in the long run. Developers that use JavaScript frameworks save time and effort on endless coding and tailor these frameworks to specific engagement needs. Also, many JavaScript frameworks are open-source, too.
It shows high-speed performance
On the client-side browser, JavaScript provides excellent performance and speed. We can quickly render an animation due to the server-side processing, ultimately enabling a seamless user experience.
It's fullstack-friendly
In JavaScript, developers can write both frontend and backend code. The MEAN stack of JavaScript (acronym – MongoDB, Express.js, Angular.js, Node.js) enables a seamless fullstack development.
It enables interoperability
Developers can use JavaScript to create various apps because this language pairs nicely with other programming languages.
It improves cybersecurity
Businesses need good cybersecurity, above all else, to protect their sensitive business data and user data. Developers can efficiently implement many helpful cybersecurity features that JavaScript frameworks offer.
It enables easy testing and debugging
It's easy for developers to recognize bugs early on because JavaScript is an interpreted language, and they can review the code by subsequent lines.
It's platform-independent
Almost every browser understands the JavaScript code quickly because this is an interpreted language and runs on all platforms or browsers.
It's excellent for creating well-designed interfaces
The JavaScript frameworks are the best choice if you want developers to create captivating interfaces, enabling a visually appealing and seamless user experience.
It has a broad developer support community
Like most popular programming languages, JavaScript has a broad community of excellent developers worldwide, always ready to share novelties, updates, or helpful development resources and tips.
Diamant sums up the beneficial aspects of JavaScript that every business owner needs to know:
1. Cross-platform compatibility – JavaScript can develop web, mobile, and desktop applications while reusing most codebases.
2. Interactive User Interfaces – JavaScript empowers businesses to create dynamic and interactive UI with real-time updates, animations, and transitions.
3. Rich web applications – We include frameworks of JavaScript, like React, Angular, and Vue, that allow businesses to build great-looking, feature-rich web and mobile applications.
4. Fast development – JavaScript's versatility, extensive libraries, and frameworks expedite development cycles, leading to a quicker time to develop features for your application.
Conclusion
Hiring skilled JavaScript developers is more crucial than ever. Whether you're creating a dynamic website or a sophisticated application, having the right talent can make all the difference.
By understanding the essential and nice-to-have skills, leveraging modern hiring strategies, and asking the right interview questions, you can find developers who meet your technical needs and bring innovation and efficiency to your projects.
As JavaScript continues to evolve, staying ahead in your hiring practices ensures your team has the expertise to tackle future challenges and opportunities.
7 things to know about JavaScript
JavaScript is a general-purpose programming (scripting) language. Together with HTML and CSS, JavaScript forms the backbone of frontend web development. It defines the behavior of dynamic web page elements done automatically in response to user actions. The JS code can be executed by JavaScript engines built into browsers or server-side JavaScript runtime environments.
What is JavaScript used for?
What is Vanilla JavaScript?
What are JavaScript frameworks?
What is fullstack JavaScript?
What are the main features of JavaScript libraries?
JavaScript libraries you must know
Compatible languages with JavaScript
Is JavaScript still widely used?
Find your JavaScript developer with your personal matcher
Get paired with your own personal matcher from our dedicated team. Why? They listen carefully to you, and can handpick the best JavaScript talent for your needs according to their skill set and culture fit.
Meet my personal matcher"With Proxify, we’ve been able to grow and work in a more flexible and creative way."
Sange Lee
Executive Vice President at Education First
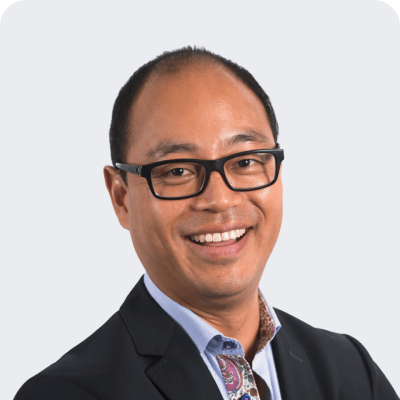
Have a question about hiring a JavaScript developer?
How much does it cost to hire a JavaScript developer at Proxify?
How does the risk-free trial period with a JavaScript developer work?
Can Proxify really present a suitable JavaScript developer within 1 week?
How does the vetting process work?
How many hours per week can I hire Proxify developers?