Hire fast with Proxify
Hire Node.js developers, fast
We know that finding the perfect Node.js developer can be time-consuming and expensive. That's why we've created a solution that saves you time and money in the long run.
Our Node.js developers are vetted and tested for their technical skills, English language proficiency, and culture fit aspects to ensure that we provide you with the perfect match for your engagement. With our hiring experts, you can easily discuss any issues, concerns, or onboarding processes and start your engagement quickly.
Our Node.js developers are also skilled in a diverse range of additional frameworks and tools, meaning you find the right candidate for your business needs, committed to delivering outstanding results, always.
Talented Node.js developers available now
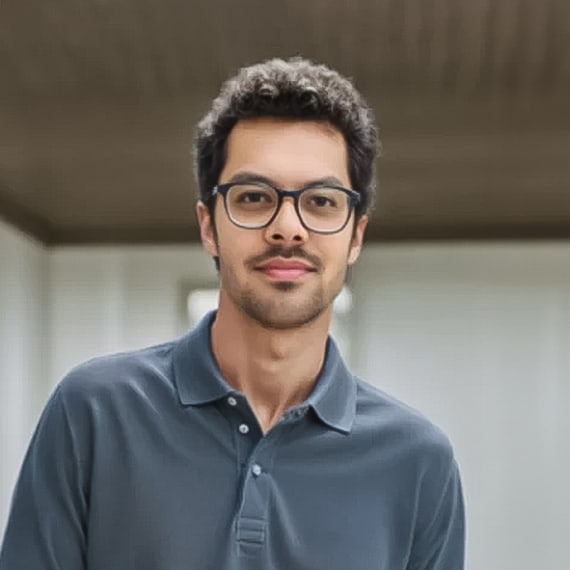
Jerome V.
Brazil
Backend Developer
10 years experience
Verified member
Expert in
- Node.js
- AWS
- C
- JavaScript
- MongoDB
- +15
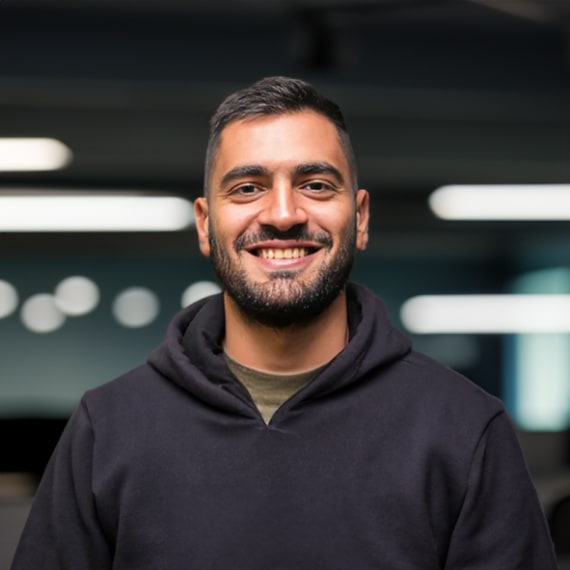
Tomas N.
Argentina
Fullstack Developer
5 years experience
Trusted member since 2022
Expert in
- Node.js
- React.js
- JavaScript
- Git
- MongoDB
- +18
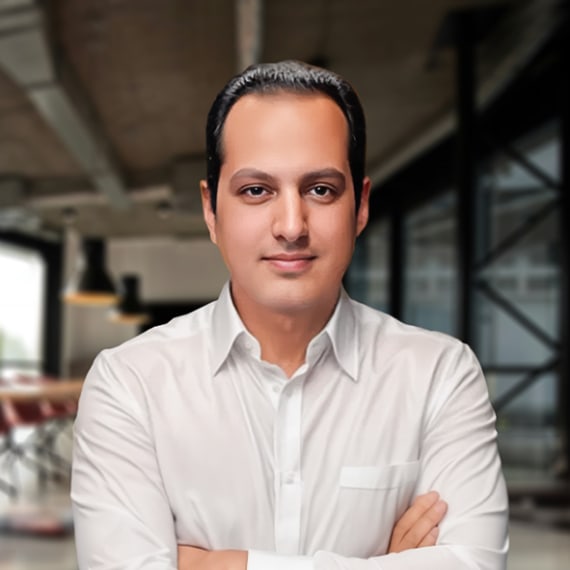
Abdelraheem J.
Jordan
Node.js Developer
8 years experience
Trusted member since 2021
Expert in
- Node.js
- Express.js
- MongoDB
- MySQL
- React.js
- +28
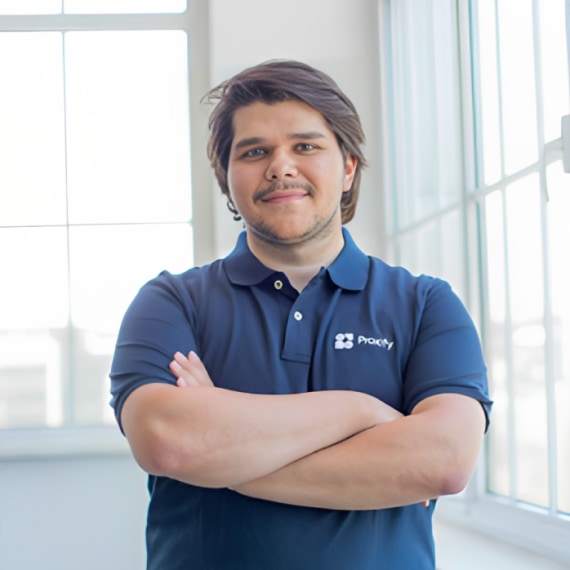
Ahmet H.
Turkey
Fullstack Developer
5 years experience
Trusted member since 2022
Expert in
- Node.js
- Vue.js
- MySQL
- Dart
- Flutter
- +16
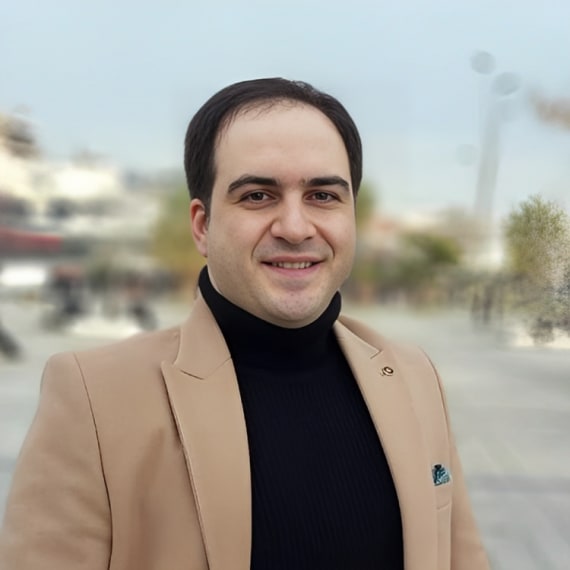
Hamed Z.
Turkey
Fullstack Developer
18 years experience
Trusted member since 2022
Expert in
- Node.js
- Angular
- TypeScript
- .NET
- .NET Core
- +8
Complete hiring guide for Node.js Developers in 2024
Node.js is a free-to-use backend solution and a runtime environment that enables the execution of JavaScript on the server.
It is famous for its remarkable performance, and it's a versatile, open-source server environment for backend programming. It is popular for businesses that work with lambdas or microservice architecture. It was released in 2009, or 13 years after releasing the first-ever server-side JavaScript environment.
Developers use Node.js on Windows, macOS, Linux, Unix, and other platforms, and predominantly for creating scalable apps.
Sourcing and interviewing a Node.js developer
To succeed at hiring a Node.js developer, you need a roadmap for each hiring stage. You also need a job ad that captures all the essentials of the Node.js developer role.
We talked to Alexandre Brindizzi, a FullStack Developer, to better understand why you should hire a Node.js developer. Some of the benefits include:
- Node.js and its ecosystem quickly evolved to meet industry requirements
- It has multiple Node.js frameworks that make it easy to use for developers
- It has a vast community worldwide, which makes it easy to source and possibly hire Node.js developers
He adds:
"A developer who knows Node.js may also know other frameworks (such as Vue.js) based on the JavaScript programming language, such as React.js or Angular. These are all factors assuring you sourced someone who will give you a full-scale architecture perspective and good practices along the development."
What does a Node.js developer do?
The primary responsibility of a Node.js developer is to deploy and manage network apps written in JavaScript or its supersets derivatives (e.g., TypeScript and CoffeeScript). They'll also create backend components, accept user data to store it in databases, and link apps to external web services. Additionally, Node.js developers work closely with frontend developers by helping them incorporate web-based programs.
Other everyday tasks and responsibilities of a Node.js developer are:
- Install and monitor each server-side network component
- Write tested, reusable, and effective code to create high-performance apps
- Ensure top-notch database performance and frontend request responsiveness
- Stay informed on Node.js trends and news
- Collaborate with frontend developers on integrating components
- Enforce safety procedures, data security practices, and storage options
- Run tests to ensure application quality
- Describe application architecture, including database schemas
- Advise, suggest, and put into practice process and technology changes
Required technical skills for Node.js Developers
Here are the technical skills to check during recruitment and interviewing. The more you tick off from this list, the better.
- Excellent knowledge of Node.js
- Broad understanding of web application principles
- Ability to write clean, efficient code
- Understanding how servers work, both Unix and Windows;
- Good knowledge of containerization solutions, such as Docker and Kubernetes, including networking between containers and pods
- Experience creating backend services (for example, in AWS technologies out of many others – e.g., deployment to Kubernetes cluster, creating lambda function, disposing of it in API gateway, etc.)
Preferred technical skills for Node.js Developers
Most of these are good to have but not mandatory:
- A degree in Computer Science or a related field
- More than three years experience if you need a mid-level developer, or more than five years experience if you need a senior developer
- Solid knowledge of frontend development
- Solid knowledge of database management (familiarity with SQL, NoSQL, MySQL, PostgreSQL, Redis, or MongoDB
- Understanding HTTP protocols and RESTful API principles
- Expertise with Version Control systems, such as Git
- Knowledge of build tools, such as Gulp or Webpack
- Knowledge of CI/CD (Continuous Integration / Continuous Deployment)
- Good understanding of server deployment
Interview questions and answers
Andrey Kolosay, a Fullstack Engineer, recommends using these questions to assess a Node.js developer candidate.
1. What is an event loop?
Example answer: Despite JavaScript being single-threaded, the event loop makes it feasible for Node.js to execute non-blocking I/O operations by offloading tasks to the system kernel when possible. Most of these contemporary kernels support several background operations because they are multi-threaded. This is precisely how an event loop operates.
2. Can you explain the central concept of Express.js in the context of Node.js?
Example answer: Express.js is a popular web framework created in JavaScript but hosted by the runtime environment of Node.js. This will set up your development environment and carry out typical web development and deployment chores, which are huge advantages of this framework.
3. Can you list some benefits of using Node.js streams?
Example answer: Streams in Node.js are beneficial because:
- They are memory-efficient, so there is little loading into the memory when we process data.
- They are time-efficient, and once we receive data, processing goes much faster than waiting for the complete payload transfer.
4. Can you explain the need for using Node.js buffers?
Example answer: We used to store raw data with an array of integers, but there is an alternative to this called the Buffer class of Node.js. This class corresponds to a natural memory allocation outside the V8 heap. An app can access the buffer class without importing the buffer module since it's a global class.
5. Can you explain why Node.js is single-threaded?
Example answer: Node.js is single-threaded since it uses just a single main thread to execute JavaScript code. It also manages asynchronous tasks through its event loop. This way, concurrency is handled well by employing event-driven architecture and non-blocking I/O operations, allowing for concurrent processing of multiple tasks. All of this means there is no great need for traditional multi-threading.
6. What are asynchronous requests?
Example answer: These requests take time to be executed, and while they request for this time, the main thread of the main loop cannot be blocked. This means an extra thread in the thread pool scales up the app.
7. What is a main loop in Node.js?
Example answer: The main loop is when Node.js coordinates what happens internally, representing the primary logic in a program. The loop can never be broken or stopped, or the app will fail. A request must always be received, confirmed, executed, and returned in the same order.
8. Can you explain the asynchronous and non-blocking APIs?
Example answer: Every Node.js library has asynchronous APIs, and at the same time, they are also non-blocking. On the other hand, a based server in Node.js doesn't wait for data to be returned by an API. Instead, the server calls the following API and goes to it. When this happens, a notification mechanism from the event of Node.js responds directly to the previous API call server.
9. What is the reactor pattern?
Example answer: This pattern belongs to the I/O non-blocking operations and functions as a handler for each I/O operation. Once we generate an I/O request, we submit it directly to the demultiplexer. This reactor pattern is the core of the asynchronous nature of Node.js.
10. What is the middleware concept in Node.js?
Example answer: The Middleware functions always have access to a request and response object and the function next in line in the app's cycle for requests and responses. This function also performs the following:
- Updating and modifying request and response objects;
- Executing code;
- Invoking the next middleware of the stack;
- Finishing the cycle of request-response.
Recognizing and selecting the best Node.js developer
To easily differentiate between good and great Node.js developers, you need to rely on the results of their technical tests.
Then, Alexandre also adds the following criteria that great Node.js developers meet:
- A proven grasp of the strongly typed and documented code
- Guaranteeing code functionality when required
- Rendering unit-testing
"They need to understand server-side and to know that containers are a good way to scale and isolate Node.js applications."
He also adds how it's vital that the developers understand the microservices principles well and always make them independent from each other. In some cases, they'll need to handle manual scaling with specific libraries where you can control the threads you scale up.
The shortlisted candidates should also be notably good at understanding application performance, especially with the Big O notations (mathematical notations that describe a function's limiting behavior when an argument leans toward some infinity value). Also, they need to work smoothly around encapsulation and preferably know how to design a complete backend.
Expertise and niches of Node.js Developers
Regarding the specializations Node.js developers can have and niche industries in which their skills are applicable, Alexandre has an ambiguous answer.
"It depends on the developer. Node.js is nowadays used for backend services and microservices. The usage of modules in JavaScript makes it very versatile in that you can also make it useful to lambdas for serverless methods."
He reaffirms that candidates for a Node.js position will probably be multiskilled fullstack developers with React.js or Angular knowledge, creating full-scale applications and using Node as complementary.
Possible challenges during the hiring of a Node.js Developer
Some common challenges you should know about when hiring a Node.js developer are
- No roadmap – Doing it all without a roadmap will make it difficult to document every hiring stage or prepare for possible setbacks. Consider a strategy before you start, and have some interview questions ready, too.
- Difficulty finding qualified candidates – The shortage of developers in recent years is familiar to anyone in the tech industry, and often, the best developers are already hired elsewhere, likely at competitors' companies. The silver lining in such situations is that there are services that vet and hire the best developers for you in just days.
- Budget limitations – Set aside enough budget for everyone involved in the recruitment and hiring and some extra budget in case the process takes longer to finish.
Business benefits of using Node.js
These are the business drivers to consider before you use Node.js.
It increases productivity
Developers save much time that would otherwise be spent switching between languages in various contexts. Besides, using JavaScript for frontend and backend is another significant advantage.
It is reusable
The versatility of JavaScript for frontend and backend goes a long way, especially with its frameworks, Meteor.js, Nest.js, and Express.js. A great Node.js developer will know when and how to choose between both.
It is user-friendly
Coding knowledge is required to work with Node.js. This is especially important for beginners and startup developers.
It is for cross-platform apps
Node.js is compatible with many platforms, such as mobile, laptop, and desktop. Developers use Node.js to make mobile apps and SaaS websites easily.
It has a large community
A large community is helpful for developers who find various solutions and assistance there. It is always active, adds and shares valuable packages, and shares actual information with everyone.
It speeds up the idea-to-product
You save time and money with a shorter development time, which is a central business driver for everyone.
It simplifies library usage for caching
Caching allows code and data storage in the app's memory database. In this context, the user response time will decrease, and the user experience will become smoother and more enjoyable.
It boosts scaling
Developers can efficiently scale using Node.js by adding consequent modules and enlarging them. The system will become easier to use and more scalable at the same time. This is mainly because, with Node.js, developers can add web apps in real-time.
It is affordable
Last but not least, affordability is another benefit of Node.js. Because Node.js uses JavaScript, companies can save by hiring fewer developers who can juggle between these two, which pays off in the long run.
Why use Node.js?
Node.js is a cross-platform JavaScript runtime environment that provides an infrastructure for running JavaScript code on the backend (server-side). It’s based on Google’s V8 engine that compiles JavaScript to machine code and ensures its super-fast execution. Node.js has an event-driven architecture and leverages asynchronous programming. Those features allow Node.js servers to process huge numbers of I/O requests coming from multiple clients at an unbeatable speed.
How to use Node.js?
What is a Node server?
Why use Node.js?
Who uses Node.js?
How secure is Node.js?
What is Node.js not good for?
What is unit testing in Node.js?
Most common Node.js testing frameworks