Builders: The podcast where we discuss the ups and downs of building great tech products Stream here
Hire senior and proven Laravel developers
Gain access to 3000+ experts, available to start work immediately.
Discover the top 2% who have passed extensive assessments.
Hire Laravel developers without additional employment fees or overheads.
Partner with a personal matcher and find Laravel developers that fit your needs.
Find a Laravel developer
Get StartedFind a Laravel developer
Get StartedHire fast with Proxify
Hire Laravel developers, fast
We know that finding the perfect Laravel developer can be time-consuming and expensive. That's why we've created a solution that saves you time and money in the long run.
Our Laravel developers are vetted and tested for their technical skills, English language proficiency, and culture fit aspects to ensure that we provide you with the perfect match for your engagement. With our hiring experts, you can easily discuss any issues, concerns, or onboarding processes and start your engagement quickly.
Our Laravel developers are also skilled in a diverse range of additional frameworks and tools, meaning you find the right candidate for your business needs, committed to delivering outstanding results, always.
Talented Laravel developers available now
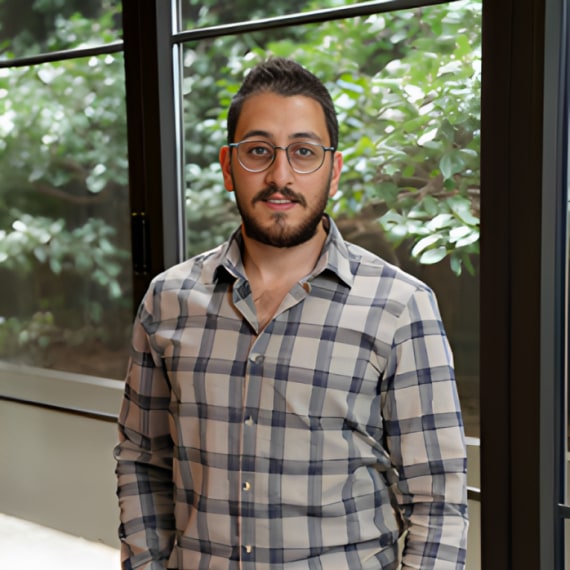
Ahmed M.
Turkey
Fullstack Developer
8 years experience
Trusted member since 2022
Expert in
- Laravel
- PHP
- MySQL
- MVC
- HTML
- +16
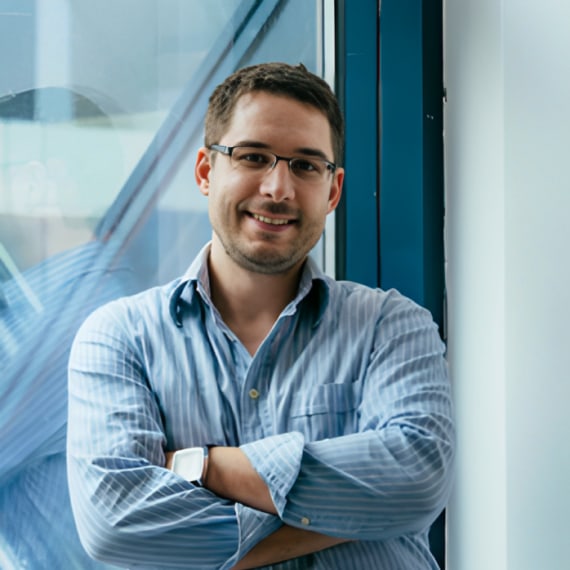
Nikola K.
Serbia
Fullstack Developer
16 years experience
Trusted member since 2022
Expert in
- Laravel
- PHP
- JavaScript
- WordPress
- Vue.js
- +19
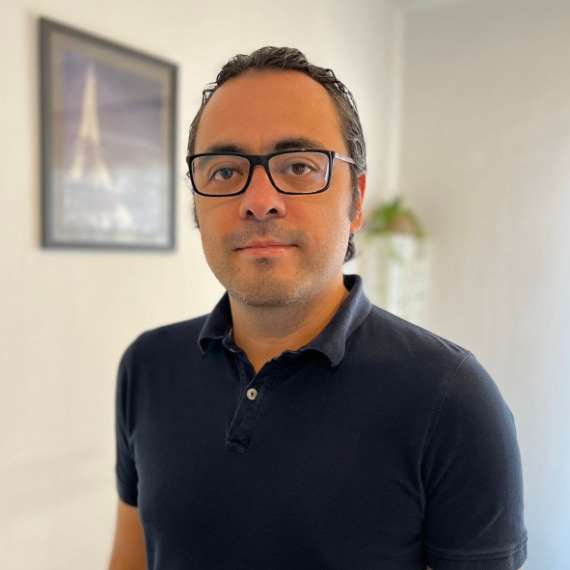
Fernando M.
Mexico
Fullstack Developer
14 years experience
Verified member
Expert in
- Laravel
- MySQL
- PHP
- TypeScript
- Vue.js
- +10
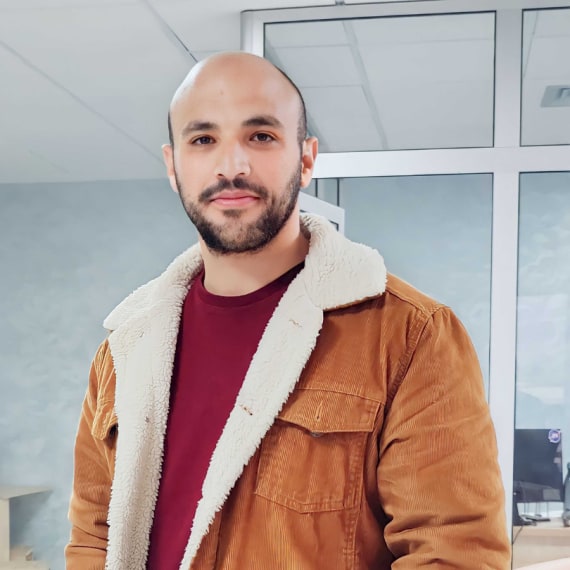
Anass E.
Morocco
Fullstack Developer
12 years experience
Trusted member since 2021
Expert in
- Laravel
- PHP
- Node.js
- Vue.js
- Shopify
- +22
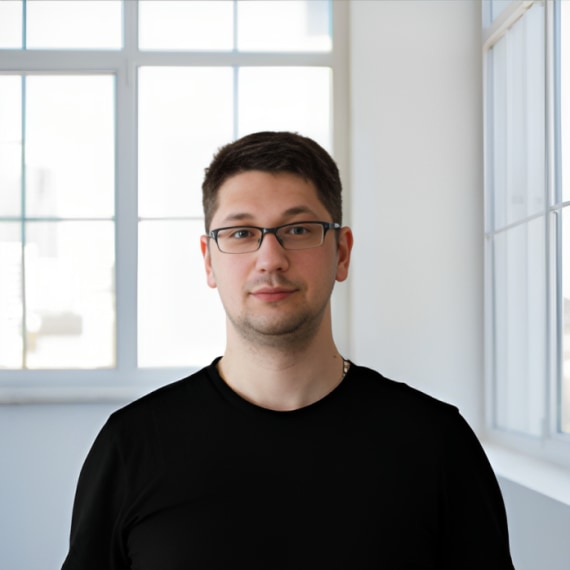
Oleg O.
Czech Republic
Backend Developer
15 years experience
Verified member
Expert in
- Laravel
- PHP
- Magento
- jQuery
- Bootstrap
- +10
How to hire Laravel Developers in 2024
As web applications become more complex, using a framework that offers ease of use and flexibility is crucial. Over the past decade, Laravel has emerged as one of the most popular PHP frameworks due to its elegant syntax and extensive feature set.
Laravel is used by many startups and enterprises around the world because of its simple yet powerful nature. The framework has also inspired many developers to create their versions based on Laravel, such as Lumen, Spark, and Silex. However, sourcing top talent in this niche technology can be challenging.
One of the biggest challenges companies face when hiring Laravel developers is finding the right person for the job. There are so many Laravel developers out there, and finding someone who is a good fit for your company can be difficult.
In this guide, we'll look at some crucial steps in hiring Laravel developers. We'll also discuss some things you should avoid when hiring a new developer so that you don't end up with someone who isn't going to work out well in your organization.
Technical skills to review when hiring a Laravel Developer
Laravel, being a powerful and versatile PHP framework, demands a specific set of technical skills to ensure efficient development, security, and scalability. Beyond a strong cultural fit and a passion for problem-solving, it's essential to delve into the technical aspects of a developer's skill set. Joshua Adebayo, a Software Engineer at Proxify, lists these skills as the foundation for building robust and high-performing Laravel applications.
-
PHP: A good knowledge of PHP programming is essential for every Laravel developer since Laravel is built on PHP. Laravel developers must understand Object Oriented Programming (OOP), Dependency Injection, Object visibility, and other critical language concepts.
-
Database: Laravel developers are required to know at least one relational database. Laravel supports Object Relational Mapping (ORM), a wrapper for interacting with databases. Having a good knowledge of databases helps to work with ORM efficiently.
-
Dependency manager (Composer): Laravel developers should understand how to add, update, remove, and version packages using Composer. It is important to note that Laravel uses Composer for dependency management.
-
Model View Controller (MVC) architecture: The MVC architectural pattern is a foundational pattern used in the Laravel framework; therefore, developers need to understand how MVC works.
-
Knowledge of cloud computing and Linux OS: Knowledge of Linux systems helps developers manage servers for hosting, configure NGINX, Apache Server, etc.
-
Design patterns: Laravel developers should know design patterns because there are a lot of them used in the framework, and also in compliance with clean code principles, e.g., repository patterns, adapter patterns, bridge patterns, etc.
Joshua believes these are the fundamentals that all Laravel developers should possess.
When asked about some of the most effective ways to assess a candidate's problem-solving abilities during the hiring process? Joshua suggests the following:
Technical interview: When hiring developers, especially Laravel developers, assessing them based on their knowledge of the language is very important. Asking framework-related questions helps understand how much the developer understands the language and the framework.
Problem-solving skills: Creating a simulation environment to test how fast developers can think and solve a problem. Platforms like Codility, TestGorilla, etc., can achieve this.
Take-home assessment: Assigning tasks to developers may be a simple engagement to understand their thought process when solving a problem, their previous experience, and how they structure their code in adherence to and compliance with coding standards.
How important is previous experience with Laravel when hiring a developer?
When hiring a developer for web application development, especially in PHP-based frameworks, the importance of previous experience with Laravel often takes center stage.
Evaluating a developer's prior experience with Laravel is critical; however, Joshua, who has personally used Laravel in the SaaS, FinTech, HealthTech, IT, and Entertainment industries, says that one must consider the nature of the role before making a decision.
"It mostly depends on the role that the person will be filling. If it's an internship role, previous experience is not required because the framework is easy to work with and has utilities that can get developers up and running. But other positions, other than an internship position, require previous experience. Previous experience helps developers gather project requirements, debug issues, effectively utilize framework features, and introduce customizations to the framework."
Joshua Adebayo
Different types of Laravel developers
There are several types of Laravel developers. Some work independently, while others work at an agency or as part of a team. The kind of developer you choose will depend on what task you need to complete and whether you need them to provide ongoing support after completion.
Laravel developers who specialize in backend development
Laravel developers focused on the backend are proficient with Laravel and can develop applications on it. They are familiar with all aspects of Laravel, including routing, view rendering, controllers, and much more. They can develop small or large-scale applications using Laravel.
Laravel developers who specialize in frontend development
They are proficient in all aspects of frontend development, including HTML5, CSS3, and JavaScript. They can help build web pages by using these technologies with Laravel. This helps to create a responsive user interface for your application that works seamlessly across multiple devices (from mobile devices to desktop computers).
Laravel developers who specialize as Fullstack Developers
These developers have expertise in both the worlds of backend and frontend development, which makes them highly sought after by companies that need help building complex applications quickly and efficiently.
Laravel-specific interview questions and example answers
During interviews, ask Laravel-specific questions to test candidates' in-depth knowledge of the framework. This could include questions about Laravel's architecture, routing, Eloquent ORM, Blade templating, security practices, and performance optimization.
1. Explain the differences between Laravel's Eloquent ORM and Query Builder. When would you choose one over the other?
Example answer: Eloquent ORM is Laravel's implementation of the Active Record pattern, which allows developers to interact with the database using PHP objects. It best suits simple to moderately complex database operations, where each table maps to a model class. On the other hand, Query Builder provides a fluent, database-agnostic way to build complex SQL queries. It's ideal for more complex queries or when working with legacy databases.
I would choose Eloquent when I'm primarily dealing with single-table CRUD operations and relationships between tables are well-defined. Query Builder is my choice when I need more control over the generated SQL or when dealing with complex queries involving multiple tables and subqueries.
2. What is Laravel middleware, and how do you create a custom middleware? Give an example of a situation where you might use middleware.
Example answer: Middleware in Laravel acts as a filter for HTTP requests entering your application. It can perform tasks like authentication, logging, and modifying the request or response. To create a custom middleware, you'd run php artisan make:middleware MyMiddleware
and then define your logic in the handle
method within the generated class.
An example situation where middleware might be used is for authentication. You could create a custom authentication middleware to check if a user is authenticated before granting access to specific routes. For instance, you might want to ensure that only authenticated users can access the dashboard route.
3. Explain the purpose of Laravel's Service Container and how it helps manage dependencies. Provide an example of binding an interface to a concrete class.
Example answer: Laravel's Service Container is a powerful tool for managing class dependencies and performing dependency injection. It's used to resolve classes and their dependencies, making it easier to manage the creation and sharing of objects throughout your application.
To ‘bind’ an interface to a concrete class, you can use the bind method in a service provider. For example:
// In a Laravel service provider's boot method
$this->app->bind(MyInterface::class, MyConcreteClass::class);
This code tells Laravel to use MyConcreteClass whenever an instance of MyInterface is requested. This allows for easy swapping of implementations by changing just this binding in the service provider.
4. What is Laravel Horizon, and why would you use it in a Laravel application?
Example answer: Laravel Horizon is a dashboard and queue manager for Laravel applications. It's built on top of Laravel's Queue system and provides real-time monitoring and configuration for queues. Developers use Horizon to gain insight into their queue jobs, manage queue workers, and retry failed jobs.
You would use Laravel Horizon in a Laravel application when you have a complex or critical queue system that requires in-depth monitoring, configuration, and management. For example, suppose you're processing many background jobs and must ensure high availability and performance. In that case, Horizon can help you achieve that by providing a user-friendly interface for queue management.
5. Explain Laravel's broadcasting and event system. How does it work, and what are some use cases for it?
Example answer: Laravel's broadcasting and event system allows real-time communication between the server and connected clients over protocols like WebSocket, HTTP long polling, or Redis. It works by broadcasting events to specific channels, which can be subscribed to by clients.
Use cases for this system include real-time notifications (e.g., chat applications, live updates in collaborative tools), broadcasting changes in data (e.g., live sports scores, stock market updates), and any scenario where you need to push updates to clients without them needing to refresh the page.
To use it, you define events and their listeners, broadcast the events when specific actions occur in your application, and then configure the broadcasting driver (e.g., Redis, Pusher) to handle the communication.
6. What is the purpose of Laravel's Blade templating engine, and how does it differ from other template engines?
Example answer: Blade is Laravel's lightweight and intuitive templating engine. It simplifies writing dynamic HTML by providing features like template inheritance, control structures, and custom directives. Blade templates are compiled into plain PHP code for better performance. Unlike other templating engines, Blade keeps the syntax familiar and does not introduce its own syntax, making it easy for developers to learn and use.
7. Explain Laravel's authentication system and how you would customize it for a specific project.
Example answer: Laravel provides a built-in authentication system with features like user registration, login, password reset, and session management. To customize it, you can use Artisan commands to generate the necessary controllers and views. You can also define your authentication logic in the AuthServiceProvider
and modify the authentication guard and provider configurations in config/auth.php.
For more advanced customizations, you can extend the built-in authentication classes or use middleware to add additional checks during authentication.
8. How does Laravel handle database migrations, and why are they important?
Example answer: Laravel's migration system allows developers to define and version-control the database schema structure in code. Migrations are created using Artisan commands and determine changes to the database schema, such as building or modifying tables. Migrations are essential because they make it easy to collaborate with other developers, roll back changes, and keep the database schema in sync across development, staging, and production environments.
9. What is the purpose of Laravel's Artisan command-line tool, and can you provide some examples of useful Artisan commands?
Example answer: Artisan is Laravel's command-line tool that simplifies various development tasks. Some useful Artisan commands include:
php artisan migrate
: Runs pending database migrations.
php artisan make:model ModelName
: Generates a new Eloquent model.
php artisan make:controller ControllerName
: Creates a new controller class.
php artisan make:migration MigrationName
: Generates a new database migration.
php artisan route:list
: Lists all registered routes in the application.
10. What is Laravel's dependency injection container, and why is it essential in Laravel development?
Example answer: Laravel's dependency injection container is a powerful tool for managing class dependencies and performing dependency injection. It allows developers to bind classes to interfaces or abstract classes, and when a class needs an instance of an interface, Laravel automatically provides it. This promotes inversion of control (IoC), making it easier to swap out implementations, perform unit testing, and maintain a clean and modular codebase.
11. Explain the concept of Laravel's service providers and provide an example of when you might create a custom service provider.
Example answer: Service providers in Laravel are used to bind classes into the service container and perform various actions during the application's bootstrapping process. Custom service providers are often created to integrate third-party packages or to encapsulate application-specific logic. For example, you might create a custom service provider to register a custom caching strategy, configure a mail driver, or set up a repository pattern for your application.
Checklist to hire and retain talent
To hire the best Laravel developers, keep in mind the following:
Establish a budget
Hiring Laravel developers can be expensive if you are not careful about how much you spend on them and what their hourly rate is going to be. The first thing you need to do when looking for Laravel developers is research your budget to find someone who fits within your price range and can meet all of your requirements.
Offer a competitive compensation package that reflects the developer's skills and experience. Highlight other benefits, such as opportunities for professional growth, flexible work arrangements, and a supportive work environment.
Know what skills you need from your developer
As mentioned before, there are many different types of Laravel developers out there, so you must know exactly what skills you need before starting the search process:
Frontend development skill – If you're looking for someone who can build HTML/CSS/JS pages, then they should have some experience with a CSS framework (Tailwind, Bootstrap, Material UI, etc.) and JavaScript frameworks (Vue.js, React.js, Angular, etc);
Backend development skill – if you're looking for someone who will work on your REST API, they should have some knowledge about databases (PostgreSQL, MySQL, MariaDB), Authentication and Authorization, HTTP protocols, etc.
"Defining requirements is a very crucial step in Hiring and SDLC. This helps to identify the skill level of developers needed for the role. This has to be clearly defined as it helps in decision making."
Craft a compelling job description
Write a job description that not only outlines the technical skills required but also highlights your company's culture, the importance of the role, and the exciting tasks the candidate will be involved in. This can help attract developers who are not only technically competent but also aligned with your company's values.
"Reviewing candidate's portfolios, the complexity of engagements they have worked with in the past, project relevance to the role they are coming in to fill, collaboration on engagements, and communication."
Onboarding and mentorship
Once hired, provide a thorough onboarding process that familiarizes the developer with your company's operations, tools, and projects. Assign a mentor or buddy to help them integrate into the team and address any questions or concerns.
Ensure they are continuously learning
Invest in continuous learning and development opportunities for your developers. Encourage attendance at conferences, workshops, and online courses to keep their skills up-to-date and ensure they stay engaged and motivated.
What makes the BEST Laravel Developer stand out from the other applicants?
"Aside from coding skills, there are other skills that developers must possess to stand out among other developers."
Joshua lists a few skills that can make a developer stand out:
- Problem-solving skills: Developers must think outside the box and think of multiple solutions to a problem. Developers must be able to break complex tasks down into smaller tasks.
- Attention to detail: Developers must be able to pay attention to detail, understand and diagnose problems, and propose the best solution to solve a problem.
- Debugging skills: To debug code properly, developers must have a good knowledge of the codebase and the project/product. Debugging code written by others helps developers to work efficiently.
- Understanding of latest trends and practices: Product development constantly evolves, with new technologies, frameworks, and best practices always emerging. Staying up-to-date with the latest trends is essential for web developers who want to remain competitive and deliver high-quality work.
- Adaptability: These might include client requests, technical issues, pressed deadlines, or restricted budgets. The capacity to adapt enables developers to respond to these circumstances successfully, using innovative problem-solving techniques and exhibiting resiliency in the face of difficulty.
What to avoid when hiring a new developer
The popularity of Laravel has led to a high demand for Laravel developers, which makes it difficult for companies to find the right person for the job. However, there are some things you can do to ensure that your hiring process goes as smoothly as possible.
Here are some things you should avoid when hiring a new developer:
Not knowing what you want from your developer
You should have clear expectations before you start looking for a developer. This will save both parties time and energy by ensuring that each candidate knows exactly what they're being hired for, which makes it easier for them to decide whether or not they're suitable for the role.
Trying to keep costs down at all costs
While cost is essential, it shouldn't be the only consideration when choosing a developer – especially if they're not qualified or experienced enough to do a good job. Suppose someone offers their services at a low price point because they're often desperate and inexperienced. In that case, they'll end up costing more in terms of wasted time and poor results than paying slightly more upfront and having a successful engagement.
"Offering competitive compensation is essential to attract top talent. It can help attract talents and reduce turnover."
Not asking enough questions
You need to ask pointed questions to get a sense of whether your candidate will be able to handle the job at hand. Make sure that when you ask these questions, you listen carefully to their responses so that you can judge their expertise level appropriately. Don't just ask questions; listen closely and probe deeper if necessary.
Not asking about technical proficiency
Many companies focus solely on past experience when hiring developers, but this isn't always enough information to determine whether someone will be able to do well at their job or not. You should also ask about current technical proficiency instead of solely working on how long they were at a previous company.
The best things about working with Laravel
Every developer has a framework they prefer to work with. For Joshua, it's Laravel. Why?
"Laravel has many built-in functions and helpers to help developers and startups quickly deliver an MVP. It also has support for packages that can accelerate development."
Other pros of this framework include:
Cost-effectiveness: Laravel is open source, making it easier for any startup without worrying about subscriptions or licensing costs.
Developer-friendly: Laravel promotes code reusability and elegant documentation that help developers build and solve problems faster. It also has a rich ecosystem readily available to assist developers in solving problems. This also guarantees the probability of finding developers quickly.
Security: Laravel provides security features like the ORM library that automatically prevents SQL injection, protection against Cross-Site Scripting (XSS) attacks, etc.
Support for deployment and server management: With Laravel, deployments and server management are simplified with tools such as Forge and Envoyer. This removes the headache of server management and helps developers and companies focus on development.
Laravel is one of the most popular PHP frameworks, with over a million monthly downloads. Companies like Facebook, Netflix, Dropbox, and The New York Times use it.
It's easy to see why Laravel is so popular: it offers a modern programming model with built-in tools that make everyday tasks easier. These include database migrations, templating syntax, queues, classes, dependency injection, etc.
As a framework with excellent documentation and community support, Laravel has become an attractive choice for many companies looking to build their next project on top of a solid foundation.
Why use Laravel?
Laravel is an open-source server-side PHP framework for fullstack web app development. Laravel was built on top of the Symfony framework and helps to create well-structured apps with model–view–controller (MVC) architecture.
What are the benefits of Laravel?
Is Laravel good for big projects?
Can Laravel apps scale?
Is Laravel still relevant in 2023?
What is Laravel Forge?
Why use Laravel?
What is Laravel Query Builder?
What is Laravel Sail?
Which Laravel version to use?
Find your Laravel developer with your personal matcher
Get paired with your own personal matcher from our dedicated team. Why? They listen carefully to you, and can handpick the best Laravel talent for your needs according to their skill set and culture fit.
Meet my personal matcher"With Proxify, we’ve been able to grow and work in a more flexible and creative way."
Sange Lee
Executive Vice President at Education First
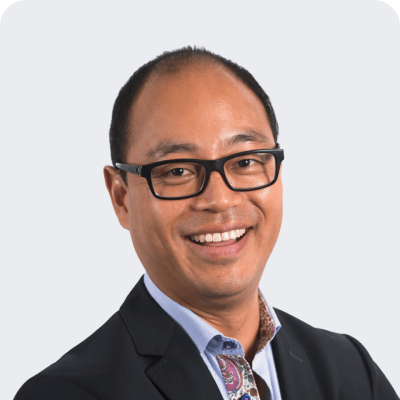
Have a question about hiring a Laravel developer?
How much does it cost to hire a Laravel developer at Proxify?
How does the risk-free trial period with a Laravel developer work?
Can Proxify really present a suitable Laravel developer within 1 week?
How does the vetting process work?
How many hours per week can I hire Proxify developers?