Hire fast with Proxify
Hire Java developers, fast
We know that finding the perfect Java developer can be time-consuming and expensive. That's why we've created a solution that saves you time and money in the long run.
Our Java developers are vetted and tested for their technical skills, English language proficiency, and culture fit aspects to ensure that we provide you with the perfect match for your engagement. With our hiring experts, you can easily discuss any issues, concerns, or onboarding processes and start your engagement quickly.
Our Java developers are also skilled in a diverse range of additional frameworks and tools, meaning you find the right candidate for your business needs, committed to delivering outstanding results, always.
Talented Java developers available now
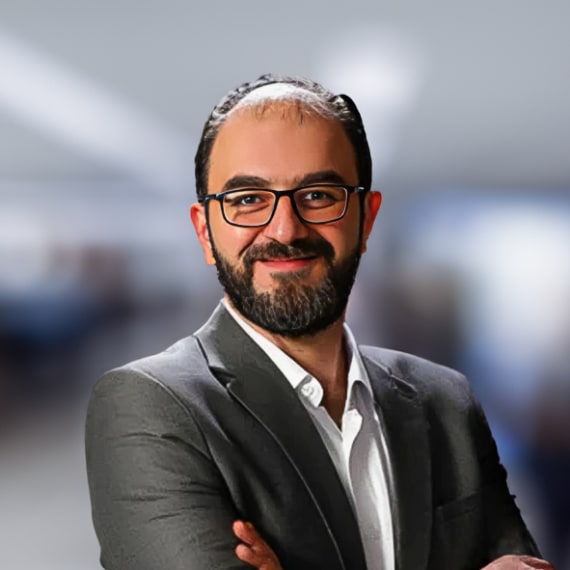
Khalid K.
Jordan
Senior Android Developer
11 years experience
Verified member
Expert in
- Java
- Android
- Kotlin
- REST API
- API
- +32
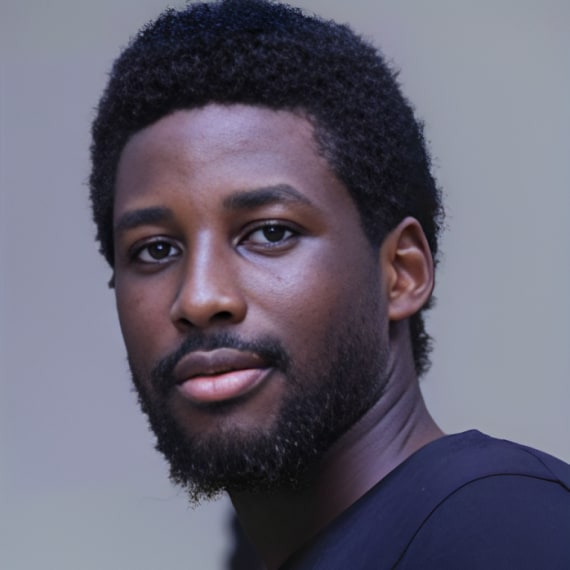
Ayezele M.
Nigeria
Fullstack Developer
10 years experience
Verified member
Expert in
- Java
- Angular
- AWS
- Hibernate
- Microservices
- +7
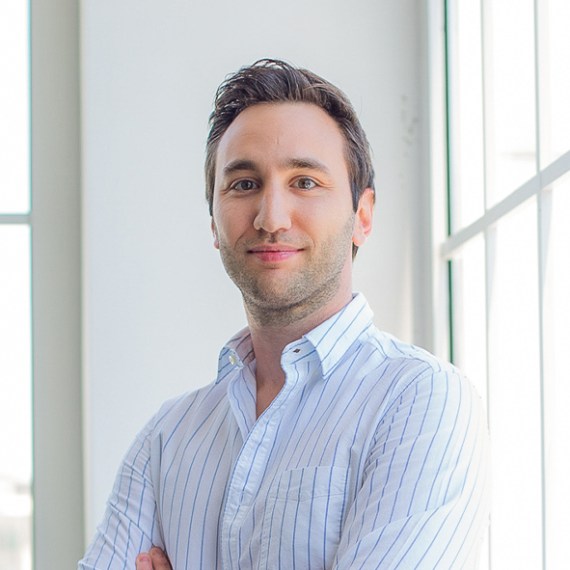
Yalın E.
Turkey
Fullstack Developer
5 years experience
Trusted member since 2022
Expert in
- Java
- Spring
- Spring Boot
- MySQL
- JavaScript
- +18
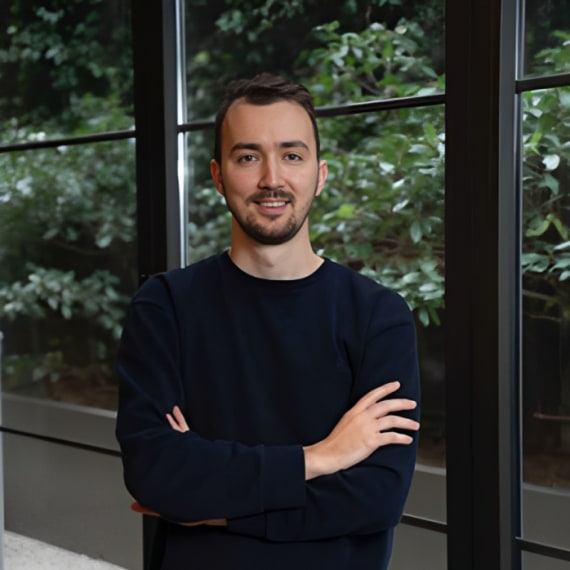
Mehmet R.
Turkey
Fullstack Developer
8 years experience
Trusted member since 2022
Expert in
- Java
- Spring Boot
- Spring
- JavaScript
- Vue.js
- +21
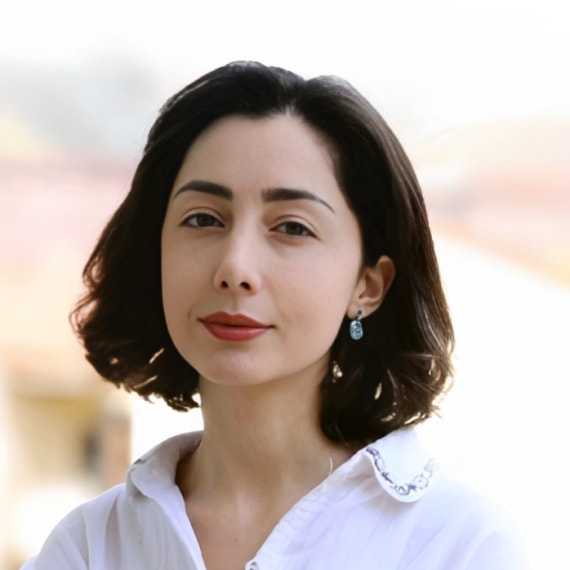
Tatev A.
Armenia
Backend Developer
9 years experience
Verified member
Expert in
- Java
- Spring
- Spring Boot
- MySQL
- Xamarin
- +17
How to find the best Java developers in 2024
The world of software development is a big playing field. It encompasses many different languages, frameworks, and other resources that sometimes make even the most experienced developers throw their hands up in defeat.
Why?
Because developers are faced with the inability to choose between all these tools, without feeling like they’re missing out on something else waiting out there. Some proverbial language/framework that is better, faster, more stable, and simpler to use compared to its competitors.
Could Java fit the bill?
Let’s find out.
What is Java?
Java is an object-oriented programming language created to have as few implementation dependencies as possible. In other words, it’s essentially an application development computing platform that is slowly but surely experiencing a second renaissance.
In terms of how it came to be, Java has an interesting history.
It was initially developed for portable devices, its creators dubbing it “OAK.” However, neither the name nor the technology took off, so project OAK was scrapped.
But, not all was lost. In 1995, Sun Microsystems (OAK’s creators) repurposed it into what is today known as “Java.” They intensely modified the programming language to ride on the emerging success of the World Wide Web.
This time, they struck gold. Java took off and is now considered one of the most popular technologies worldwide, despite the language’s relatively mature age.
Java popularity
According to PYPL, or The PopularitY of Programming Language Index, Java was ranked the 2-nd most popular programming language worldwide. It has seen just a slight drop of usage by -1.7% compared to the previous years.
The way PYPL works is it analyzes the popularity of programming language tutorials on Google search. The more search queries a given language receives, the more popular it’s assumed to be. More precisely, PYPL uses data from Google Trends.
Additionally, Java remains among the top three contenders for the most popular languages used by software engineers worldwide. The TIOBE index lists Python, C, Java, and C++ as owning exactly half of the market share (50%) out of all programming languages in use today, and Java is third by popularity in 2023.
Where to find Java developers
There are several ways to find a programmer proficient in the Java language, including:
- Word-of-mouth referrals
- Hiring agencies
- Talent-matching services
Regarding word-of-mouth referrals, this may have worked better in the past, but now things are different. People spend less time in the office, commuting, or mingling, and more time in front of their computer screens in the settings of their own homes.
The changes of this shift in working habits is obvious: more time for family and friends, increase in savings, but also a decrease in the time spent socializing outside your primary social group.
Which inevitably means that the chances of someone else recommending you in person are getting slimmer by the day. Thankfully, some social networking sites are trying to offset this trend, but for how long and whether it will leave a lasting imprint on the ways we do things, only time will tell.
The second option to get your hands on a quality Java programmer is to use an intermediary, like a hiring agency. If you go down that route however, be prepared to pay a premium fee. Hiring agencies have also been known for their tendencies to upsell, so be wary of that fact too.
Finally, if you want a continuous integration with your project, partners, clients, and developers all in one, you can opt for a talent-matching service like our own – Proxify.
We are an elite network of professional developers from multiple disciplines and subdisciplines, proficient in most of the popular languages and frameworks used in backend, frontend, and fullstack development today.
We can match you with the best developers for your needs, including those proficient in Java and notable Java frameworks, in a matter of days. If you’re not satisfied with your developer, we can expressly look for another match, or give a full refund for the first week of using our services, no questions asked.
Other popular Java talent marketplaces include Gigster, Dice, Startupers, The Muse, and more.
How to interview Java developers
Interviewing Java developers can be broken down into three stages:
- Assessing their soft skills
- Checking their technical proficiency in the language
- Testing their problem-solving abilities in real time
Working on a complex project, like a Java-based web application, usually requires more than a single developer to get it done efficiently. This inevitably creates the need for clear communication, coordination and proper code version control between each team member.
A quality Java developer will be able to communicate with their team effectively, relay new information as it arises and prioritize the parts of the application development cycle that will make the most impact in the least amount of time.
In terms of checking for technical proficiency, the responsibility is also on the technical recruiter as much as it falls onto the interviewees themselves.
A good recruiter will be able to ask the right questions, be familiar with Java, the Java platform, and most popular Java frameworks as well.
Finally, in order to test a Java developer’s problem-solving abilities fairly and properly, the technical recruiter must be able to provide real-life examples (or very good “dummy” code) of a Java project that needs to be improved, completed, or corrected in some way, shape, or form.
They would then handle this to the promising Java developer, and the dev will be able to solve the problem on the spot, or provide alternatives if the solution is too complex to be integrated during the interview part of the hiring stage.
Top technical skills Java developers should learn
It’s no secret that today’s developers are more akin to jacks of all trades (in a good way) rather than specialists with a narrow focus on a single programming language.
So, a quality Java developer will also be well-rounded in several other highly and tangentially relevant areas. These include:
DevOps: Following modern DevOps principles is a crucial skill for any Java developer, regardless of their background. They can improve communication and boost productivity all at once. Java devs proficient in DevOps best practices can speed up their code deployment process multiple-fold and create new ones significantly faster.
API skills: Knowledge of API (Application Programming Interface) development and integration has to be second nature to every developer that is serious about their craft. For Java developers, the most important APIs to know are XML processing APIs, JSON processing APIs, and Logging APIs. Out of these, some of the more popular libraries to know include JAXB and Stax.
Spring Boot: Spring Boot is essential for Java developers to be familiar with as it pairs web development with an agile approach for better product prototyping. It also provides metrics, supports JAR files, and has tons of plugins to choose from, thus reducing costs and improving project quality simultaneously.
Java skills: Java programmers have to know the foundation upon which the Java programming paradigm runs. Aspects of this include knowledge of abstract classes, as well as behavioral, creational, and structural design pattern skills.
JVM: JVM or Java Virtual Machine enables successfully executing a Java program and provides a runtime environment for developers to run Java bytecode.
Pursuing this line of thought even further, I was curious how seasoned developers were implementing Java in their everyday routines. Here were my three questions:
- What is Java best used for?
- What is project Loom and how will it help Java developers moving forward?
- Do you personally think Java will someday go out of fashion? If yes, what is the most likely language/framework to replace Java?
Koray Gecici, an expert developer from the Proxify network, shared his thoughts on my inquiries.
He said that Java is best used for backend development. “There are lots of open source libraries that make it easy to develop scalable and reliable backend applications, including microservice architecture”, Koray pondered.
Regarding project Loom, here’s what Koray had to say.
“In general, concurrency is hard for developers. When we think of today's transaction counts, the traditional threading model is not enough to handle millions of requests. To overcome this problem, we are developing using the microservice architecture and in case of load, we are able to increase nodes. But this approach is costly according to the model that Loom offers. With the same amount of CPU reducing context switching, we will be able to handle more requests per transaction.”
How about future-proofing the already “ancient” Java language? Is there any merit to the notion that Java will eventually go out of fashion?
Koray ended with a bang. “In the long run, yes. But since there is a huge existing codebase, developers will be able to find jobs”, he laughed. “Like mainframe”, Koray laughed again.
“Python and various AI frameworks would (eventually) replace Java”, Koray concurred.
Meanwhile, another developer was also ready to chime in on the matter. Yusuf Yigit is a senior software developer specializing in Java, Spring Boot, Microservices, and more languages/frameworks.
“Java is a very capable and broadly used programming language. JVM makes the Java platform independent. In this way, Java can be used for creating almost every kind of application, from microcontroller IoT to large enterprise systems.”
He then proceeded to shed some light on Loom, the project that looks to upgrade Java to modern-era programming.
“Threads are the essential parts of the Java concurrency world. To this day, before project Loom, Java threads were using comparably more resources to work in the operating system. With project Loom, threads will become more lightweight and consume fewer resources. This will result in a high-throughput, lightweight concurrency model in Java”, Yusuf said.
But, is Java going out of fashion? Yusuf doesn’t think so:
“In the near future I don't expect any other language to replace Java, or more precisely other JVM languages. As a high level language and a good evolution of the framework (beside backward compatibility), Java will remain the most used language worldwide. But I am thrilled by newly created languages like Go and Rust.”
Top soft skills Java developers should have
The most important soft skills a Java developer should have are as follows:
Communication: Being able to successfully summarize different ideas and communicate them to their coworkers, superiors, and clients is the number one soft skill a prospective employee should have.
Bad communication reduces the effectiveness of people, reduces productivity, and leads to unwanted friction between team members down the road. If you’re hiring Java developers, especially project leads, then picking someone with good communication skills is vital for the project's future success.
Organization: A highly skilled Java dev should know the importance of good organizational abilities. Mostly this would translate into writing and documenting quality code, including working with the most popular version control systems GitHub or GitLab. Sometimes, however, candidates will need to combine these resources with other project management tools to facilitate their workload in an efficient manner.
Working in a team: People that jibe well can do much more than those who always bicker with each other. Needless to say, teamwork is an important skill that quality Java developers should have mastered by the time they are ready to tackle bigger projects.
Problem-solving abilities: Developers solve problems daily, so they should be naturally good at it, right? It’s wrong to assume that all software developers are good problem-solvers, and this is why you should facilitate a real-life test during the interview with your Java devs. Being able to “think outside the box”, or even “become the box” if need be (Metal Gear, anyone?), is very much required if developers want to go from being “good” to becoming “stellar”.
Adaptability: New Java versions are constantly being deployed, so great Java devs need to keep up with this rapid tempo by being adaptable and ready to change. They should also be ready to differentiate between different Java versions with ease, and point out errors in the code pertaining to different versions of the project. Being adaptable prepares them for the unexpected, which, honestly, happens more often than developers and project leaders would like to admit.
Java interview questions and answers
Getting the right people in the right positions can make or break your business. Java developers will have years of experience dabbling in Java applications, as well as tons of time put into developing cloud computing apps and other projects that would’ve made them learn Java.
But, how do you know if they are as technically well-founded as they claim to be? By asking them Java-specific questions.
Here are some:
1. What are the main features of Java?
Expected answer: Java is object-oriented, portable, platform-independent, dynamic, distributed, and it features a relatively simple syntax that upcoming developers can learn in a matter of weeks.
2. How is the main()
method used in Java?
Expected answer: In Java, main()
method is considered the entry point for any Java program. The full syntax is as follows: public static void main(String args[])
3. How many access modifiers does Java feature?
Expected answer: There are four access modifiers. These include public, protected, private, and default.
4. What function does the volatile keyword serve in Java?
Expected answer: The volatile keyword is used with variables in Java. The threads read the volatile keyword value from the memory location, thus avoiding caching. Using the volatile keyword ensures that both the read value and the memory location are identical.
5. When does the OutOfMemoryError
appear in Java?
Expected answer: OutOfMemoryError
is considered a subclass of java.lang.VirtualMachineError
and appears when the JVM runs out of heap memory. One solution could be to add more memory in the JVM with the following options: $>java MyProgram –Xms1024m –Xmx1024m –XX:PermSize=64m –XX:MaxPermSize=256m
Why should you hire a Java developer?
Java follows the “write once, run anywhere” paradigm, meaning that you can use the same Java code on multiple devices and operating systems likewise.
Additionally, you can also reuse entire Java applications for cross-platform development purposes, where code reusability can save your business tons of cash in the long run.
To top it all off, Java has been proven time and again to be secure, flexible, robust, and reliable. The Java system is built as such to seek vulnerabilities within the code in a closed environment, automatically safeguarding the system against any external attacks. Industries such as Healthcare, Finance and Education have lots to gain from integrating Java as their preferred language of choice.
Having top Java developing talent, you’ll make sure your applications are set to adapt against any changes in the future. This can help your organization thrive, grow and scale as the marketplace demands.
What is Java?
The Java programming language is an open-source application development platform first developed by Sun Microsystems in 1995. The three most important principles of Java include inheritance, encapsulation, and polymorphism. Inheritance means that code can be reused between classes and subclasses. Encapsulation is a security principle that prevents any outside users from changing any parameters in any class. It can be set to public, private, or protected. Finally, Polymorphism means that one interface can be used to perform multiple actions at the same time.
Why use Java?
Most used Java frameworks
Biggest benefits of using Java
Common uses